Creating Smooth Animations with Tween Animation in Flutter

Introduction
Animation is an essential component of any user interface, and Flutter provides a powerful animation framework that allows developers to create beautiful and engaging animations with ease. One of the key components of this framework is the Tween class, which enables developers to interpolate between two values over a specified duration. In this blog post, we’ll explore how to use Tween animation in Flutter to create engaging and dynamic user interfaces.
Read other articles on flutter here.
What is Tween Animation?
Tween animation is a type of animation in which a property of a widget is changed over time. The Tween class provides an easy way to interpolate between two values, making it ideal for creating animations in which the starting and ending values are known.
In Flutter, the Tween class is part of the animation library and can be used to animate a wide range of properties, including colors, positions, sizes, and opacity. When using the Tween class, you define the starting and ending values of the property you want to animate, as well as the duration of the animation. The Tween class then calculates the intermediate values between the starting and ending values, allowing you to create smooth and visually pleasing animations.
How to use Tween Animation in Flutter
To use Tween animation in Flutter, you first need to create an AnimationController object. This object is responsible for controlling the animation’s duration and playback. You can specify the duration of the animation in milliseconds or seconds, depending on your requirements.
Once you have an AnimationController object, you can create a Tween object to interpolate between two values. The Tween class is a generic class that takes a type parameter specifying the type of value to be interpolated. For example, if you want to interpolate between two colors, you would create a Tween<Color> object.
After creating the Tween object, you can call the animate method on the Tween object and pass it the AnimationController object. This creates an Animation object that can be used to drive the animation.
Finally, you can use the AnimatedBuilder widget to build the user interface and update the values of the widget being animated. The AnimatedBuilder widget takes an Animation object as its argument and rebuilds the widget tree every time the animation value changes.
Here’s an example of how to use Tween animation in Flutter to animate the background color of a container:
import 'package:flutter/material.dart';
class TweenAnimation extends StatefulWidget {
const TweenAnimation({super.key});
@override
TweenAnimationState createState() => TweenAnimationState();
}
class TweenAnimationState extends State<TweenAnimation>
with SingleTickerProviderStateMixin {
late AnimationController _controller;
late Animation<Offset> _animation;
@override
void initState() {
super.initState();
_controller = AnimationController(
duration: const Duration(milliseconds: 500), vsync: this);
_animation = Tween<Offset>(
begin: const Offset(-1, 0.0), end: const Offset(1.0, 0.0))
.animate(_controller);
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
void _forward() {
_controller.forward();
} void _reverse() {
_controller.reverse();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Tween Animation Example'),
),
body: Column(
children: [
Expanded(
child: Center(
child: SlideTransition(
position: _animation,
child: Container(
width: 100.0,
height: 100.0,
color: Colors.blue,
),
),
),
),
Padding(
padding: const EdgeInsets.all(50),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
SizedBox(
width: 150,
child: ElevatedButton(onPressed: _forward
, child: const Text("Forward",style: TextStyle(fontSize: 25),)),
),
SizedBox(
width: 150,
child: ElevatedButton(onPressed: _reverse
, child: const Text("Reverse",style: TextStyle(fontSize: 25),)),
),
],
),
),
],
),
);
}
}
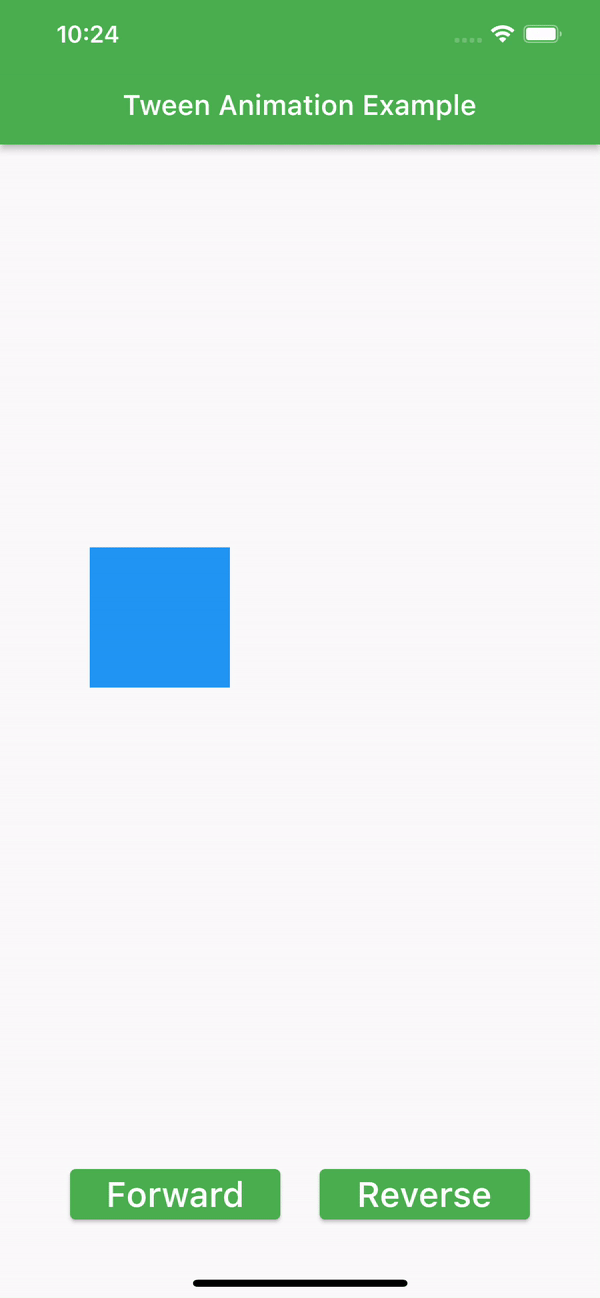
In this example, we create a Tween
object with a beginning value of
(the current position of the widget) and an ending value of Offset(-1, 0.0)
Offset(1.0, 0.0)
(the new position of the widget). We then create an AnimationController
object with a duration of 500 milliseconds and pass it to the Tween
object to create an Animation
object.
When the Forward action button is pressed, we call the _forward
method, which starts the animation by calling _controller.forward()
. Then when Reverse button is pressed we call _reverse me
thod, which reverse the animation by calling _controller.reverse()
. The widget’s position is updated by the SlideTransition
widget, which takes the Animation
object as its position
parameter.
You may have notice our TweenAnimationState is extends with SingleTickerProviderStateMixin.
What is SingleTickerProviderStateMixin?
SingleTickerProviderStateMixin is a mixin class provided by Flutter that simplifies the management of an AnimationController object in a StatefulWidget. This mixin class provides a TickerProvider object that can be used by the AnimationController to schedule animation frames.
When using SingleTickerProviderStateMixin, a single AnimationController can be managed with ease, allowing developers to easily create and manage animations in their apps. It simplifies the process of starting and stopping an animation and ensures that the animation is properly disposed of when the StatefulWidget is removed from the widget tree.
Conclusion
In conclusion, the Tween class is a powerful tool for creating animations in Flutter. By allowing developers to interpolate between two values over a specified duration, it makes it easy to create smooth and visually pleasing animations. In this blog post, we’ve covered the basics of using Tween animation in Flutter, including how to create an AnimationController object, a Tween object, and how to use the AnimatedBuilder widget to build the user interface.
With the Tween class, Flutter developers have the power to create engaging and dynamic user interfaces that are sure to impress users. We hope that this blog post has provided you with the knowledge you need to get started with Tween animation in Flutter and that you can use it to create amazing user interfaces for your apps.